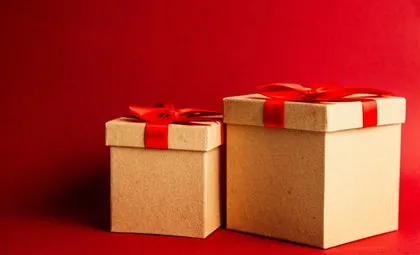
Validating Xml in PowerShell
Sometimes a deployment pipeline needs to know that a file is at least vaguely sensible before trying to use it. Here is a short script cmdlet that accepts a file path (or wildcard) and attempts to load the xml as a valid document. Any exceptions caused by document errors will show up as errors and break a build with an exit code of 1.
[CmdletBinding()]
Param (
[Parameter(Mandatory=$true, HelpMessage="Path or wildcard to xml file to test.")]
[ValidateNotNullorEmpty()]
[string]$Path
)
Resolve-Path $Path | % {
$XmlFile = $_.Path
If (!(Test-Path -Path $XmlFile)) {
throw "The file $($XmlFile) does not exist"
}
$xml = New-Object System.Xml.XmlDocument
Try {
$xml.Load($XmlFile)
}
Catch [System.Xml.XmlException] {
Write-Error "$Path : $($_.ToString())"
exit 1
}
}
Note that I support wildcard paths by using Resolve-Path to generate a list of matching paths if, for example, we run the cmdlet with *.xml input.