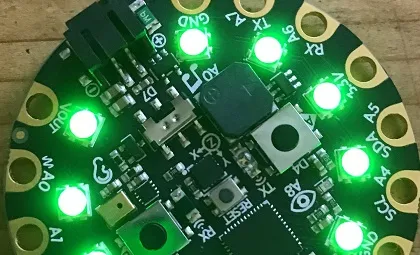
Circuit Playground Express Moods
The latest programmable gadget I've been messing around with has been the Adafruit Circuit Playground Express, a device something like the marvellous microbit but with even more sensors, neopixels built in, and it can be programmed with c++ or Python.
Mood
First off, I wanted to build an "easy" project, something visual, that would show off the neopixels built into the board. I hit on the idea of a "mood" light or a light that would cycle through the spectrum of colours, slowly shifting from one to another and cycling around indefinitely.
First
First off, the playground express doesn't come with Python pre-installed, it requires a bit of setup before that can work.
With the microbit, you can name a source file whatever you want and the editor (Mu) will handle flashing the data onto the device. Things are a bit different with the express. You have to be connected to the device through the USB and you have to save the code into the top level directory as "code.py". Saving over the top of this file will reprogram the device to the new script.
Second
Once that's done, to use the neopixels most easily, the express has a set of libraries that you can copy into the lib folder. For neopixels and colour handling, the fancyled library comes in very useful and it's what I will use in this example.
LEDs
So with that all in place we can begin writing our code. We need to import the circuit playground library to access the buttons and the express variant so we can talk to the Neopixels. The pixels are super bright by default so I tend to wind down the brightness at the start and use the a and b buttons to step it up and down as required.
HSV
To create a single colour, I elected to start with an HSV value of 0 and map that to an RGB colour space. The neopixels require that that value be "packed" into a format that it can understand. The reason for picking an HSV value was because we can smoothly iterate through all the supported colours just be starting at zero and stepping through a fractional increment each time until we eventually roll over at the maximum, 1.0
Each time round the loop we calculate a new colour and update the pixels. The step size and the time between updates is controlled by variables at the top of the program.
Code
# mood-lights.py
from adafruit_circuitplayground import cp
from adafruit_circuitplayground.express import cpx
import adafruit_fancyled.adafruit_fancyled as fancy
import time
brightness_step_size = 0.05
brightness = brightness_step_size
cpx.pixels.auto_write = False
cpx.pixels.brightness = brightness
PIXELS = 10
hue = 0.0
step_size = 0.001
time_step = 0.02
while True:
hsv = fancy.CHSV(hue)
rgb = fancy.CRGB(hsv)
pixel_colour = rgb.pack()
for i in range(PIXELS):
cpx.pixels[i] = pixel_colour
cpx.pixels.show()
time.sleep(time_step)
hue += step_size
if hue > 1.0:
hue = 0.0
if cp.button_a:
brightness = min(brightness + brightness_step_size, 1.0)
cpx.pixels.brightness = brightness
elif cp.button_b:
brightness = max(brightness_step_size, brightness - brightness_step_size)
cpx.pixels.brightness = brightness