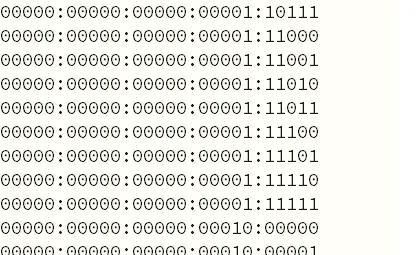
Microbit Binary Clock
I managed to find a microbit (most of them put away due to not teaching for a while) and ported over the clock from yesterday's post to run on it. Most of the code stayed the same with the main difference being the binary string is now 0s or 9s rather than 0 or 1, just so that the display would be bright enough.
from microbit import *
# rjust does not exist in micropython
def rjust(text, character, length):
padding = character * (length - len(text))
return padding + text
def decimal_to_binary(decimal, length):
binary = bin(decimal).replace("0b", "")
return rjust(binary, "0", length)
def format_binary_for_screen(binary):
formatted = ":".join(binary[i : i + 5] for i in range(0, len(binary), 5))
return formatted.replace("1", "9")
# test
# reset_value = "00000:00000:00000:00000:99999"
reset_value = "99999:99999:99999:99999:99999"
value = 0
binary = decimal_to_binary(value, 25)
formatted = format_binary_for_screen(binary)
while True:
binaryAsImage = Image(formatted)
display.show(binaryAsImage)
# print(formatted)
if formatted == reset_value:
value = 0
else:
value += 1
binary = decimal_to_binary(value, 25)
formatted = format_binary_for_screen(binary)
sleep(1000)
25 bits of counting gives 2 ^ 24 possible values, which one per second, gives us about 194 days of counting before it resets back to zero again. If the microbit had had one more bit then we could have had enough time to count out a full year.
Without knowing anything about how binary works, I think the program makes for a nice, calming display that twinkles away as it counts but does put me somewhat in mind of those old fashioned 60s computers where there would be random blinking lights flashing to show that something was happening in a high-tech way.