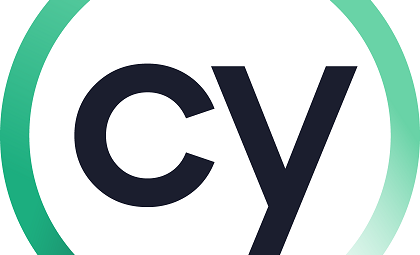
Better Date Handling in Cypress
I've been getting into using Cypress for API testing recently and needed some nicer way of handling dates than just the raw Date class in JavaScript. At this point in time - who knows it might be different by the time you read this :) - dayjs seems to be the way to go rather than moment or one of the others.
The process of how to add it to Cypress and expose it in a nice way turned out to be fairly easy. First we need to install dayjs, usually I use npm but other package managers work just as well I am told.
Once the package is installed, I needed to make Cypress aware of it. API tests come under the umbrella of end-to-end-tests so the e2e.js file under the cypress folder was the place to do that.
e2e.js
const dayjs = require('dayjs')
const utc = require('dayjs/plugin/utc'); // we handle dates in utc only
dayjs.extend(utc);
The main dayjs is required plus the utc plugin so we can work with UTC dates.
Once the utc extension has been added, we can hang dayjs on the global Cypress object.
e2e.js
Cypress.dayjs = dayjs;
and then access it in the test code like this:
it('api can do dates', () => {
const today = Cypress.dayjs.utc();
});
This works for general exposure to the full utility but we can also set up some custom functions if we want:
e2e.js
Cypress.formatted_date = () => dayjs.utc().format('YYYY-MM-DD');
it('api can do dates as text', () => {
const today = Cypress.formatted_date();
});