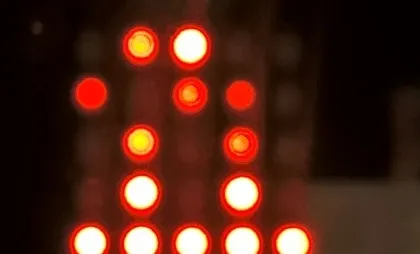
Rock, Paper, Microbit
I saw a tweet from @TechForLifeUK the other day which said they were going to run a code dojo where they would be writing the game of rock, paper, scissors on the microbit.
RPS is a simple game that I have written a number of times in different languages, usually in my ruby deliberate practice sessions, but I had never tried doing it in python and never for the microbit. Clearly that kind of challenge could not go unanswered :)
The Game
The game design I came up with was as simple as I could get it - shake the microbit and it would make a random choice between one of the three options. When it had decided on an option, it will display an image representing that choice and keep it on the display for a few seconds. After that the display will clear and you need to shake again to pick another random shape.
It's up to you to throw your own shape, compare with the microbit and keep score.
Shapes
The most challenging part of the program, as usual with developing games for the microbit architecture, is coming up with suitable images to represent game concept, in this case the rock, paper and scissors. Luckily I have a background in creating icons for Windows application but even that is not much help when the system is constrained to a 5 x 5 LED grid.
Eventually I settled on an oval shape to represent the rock, a document kind of icon to represent paper, and an extremely rough approximation of a pair of scissors.
Images
Images on the microbit are represented as a single string of numeric characters, arranged in a 5 x 5 grid with each line separated by a colon. Each number represents the brightness of one LED "pixel" - 0 is off and 9 is full brightness. For example, a square would be shown by lighting up the edges of the grid and leaving the inside of the grid dark. Like this:
Image("99999:"
"90009:"
"90009:"
"90009:"
"99999")
Clues
To provide a little bit of a clue to what the player can expect, I cycle through the three alternatives at the start of the game using display.show with the list of images.
Here's the finished code:
# Rock, Paper, Scissors
#
# Shake the microbit and it will pick
# a shape at random.
from microbit import *
import random
# Images of R, P and S
rock = Image("00000:"
"09990:"
"90009:"
"92229:"
"29992")
paper = Image("99950:"
"90992:"
"90092:"
"90092:"
"99992")
scissors = Image("90009:"
"09090:"
"00900:"
"55055:"
"55055")
rock_paper_scissors = [ rock, paper, scissors ]
display.show(rock_paper_scissors, delay = 500)
display.clear()
while True:
if accelerometer.was_gesture("shake"):
one_of_them = random.choice(rock_paper_scissors)
display.show(one_of_them)
sleep (3000)
display.clear()