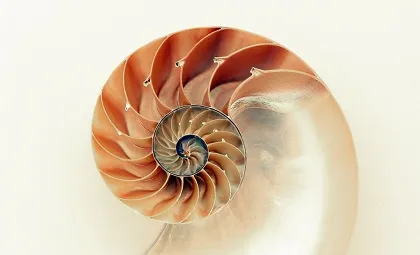
PSake Cheatsheet
PSake is a PowerShell module, similar to msbuild, that allow you to parcel up chunks of code into discrete Tasks and create dependencies between them. PSake then determines the order they should run and handles that complexity. Here are the highlights of functionality I use all the time.
Tasks
The basic unit of execution in PSake is the Task:
Task Run-FirstTask -Description 'My First Task' {
Write-Host 'Doing stuff'
}
You can give each task a description as well as a name to help with documenting what each task is supposed to do.
Running
We run the task by invoking PSake (after Importing the module if we need to):
Invoke-Psake .\MyFirstTask.psake.ps1
Invoke-Psake -BuildFile .\MyFirstTask.psake.ps1 -NoLogo -Verbose
A nice feature of running is a report at the end showing how long the process took overall and how long each individual task took.
Discoverability
PSake scripts can become complicated to read through in one go so it supports self-documenting of all of your Tasks and their dependencies using the -docs switch:
Invoke-Psake .\MyFirstTask.psake.ps1 -docs
Dependencies
More than one Task can depend on a sub-task and PSake will work out the correct order of execution to honour each dependency statement.
Task MyFirstTask -Depends CleanStuff, CopyStuff {
}
Task CopyStuff -Depends CleanStuff {
}
Task CleanStuff {
}
Default
A default task can be run if nothing is specified from the command line.
Task Default {
}
Parameters
PSake can accept parameters that it makes available as variables in your tasks to allow them to be a little more flexible:
Invoke-Psake -Parameters @{ 'Version' = '1.0' }
$Parameters {
$Version = '1.0.0'
}
Invoke-Psake -Parameters $Parameters
Alias
A task can have more than one name.
Task ? -Description 'List tasks' -alias 'Help' {
WriteDocumentation
}
Assertions and Required Variables
To add to the robustness of a script you can ask that PSake will error if one or more variables are not declared. You can also check that a condition is met inside a task by using the Assert statement.
Task Copy-Files -RequiredVariables BuildPath, Version, MachineName {
}
Task Verify {
Assert (Test-Path 'c:\scripts\debug.txt') 'Debug.txt file has not been deployed'
Assert (![string]::IsNullOrEmpty($Version)) '$Version was null or empty'
}
Preconditions
PSake will skip a Task if the precondition is not met.
Task Copy-Files -Precondition { $MakeACopyOfFiles -eq $true } {
'Making a copy of the files'
}
Task formatting
You can make individual tasks stand out by formatting the task name output. Either by setting FormatTaskName with a string or a code block.
FormatTaskName "------- Executing {0} Task -------"
FormatTaskName {
Param($TaskName)
Write-Host "------------------ $TaskName ------------------ " -ForegroundColor Green
}