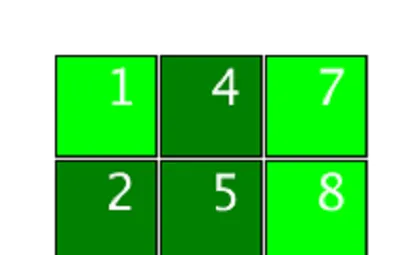
Game of Oligarchy Part 2
Finally got back around to making some enhancements to the game of oligarchy I started in processing at the beginning of the month.
I wasn't done with the game because I was just using a coin toss against every player who was not bankrupt rather than pairing them off against each other. The rules of the game call for each player to be paired with another and for each to bet the lower of the two players available stakes which amounts to half their current pot.
I needed to change the algorithm for play within the draw method but the changes weren't too difficult to implement.
Better Game
Rather than play each player every time, we start off the draw method with a list of unpaired players (the players still in the game) and an empty dictionary of the pairs we are going to build. I use random to pick each player out of the unpaired list and then remove them so we don't accidentally pick them twice.
def draw():
background(255)
# allocate players to pairs
unpaired = []
paired = {}
for p in players:
if p.is_playing():
unpaired.append(p)
# pair them off
while len(unpaired) > 1:
firstChoice = int(random(len(unpaired)))
player1 = unpaired.pop(firstChoice)
secondChoice = int(random(len(unpaired)))
player2 = unpaired.pop(secondChoice)
paired[player1] = player2
# play each pair
for p in paired:
player1 = p
player2 = paired[p]
bet = min(player1.stake(), player2.stake())
# toss a coin
pick = int(random(len(coin_options)))
heads_or_tails = coin_options[pick]
println('coin is ' + heads_or_tails)
if heads_or_tails == 'tails':
player1.win(bet)
player2.lose(bet)
else:
player1.lose(bet)
player2.win(bet)
# show each status regardless of
# whether they played
for p in players:
p.display()
I had to add in a couple of lines to work with only the paired players, agree on a bet amount and then award a win and a lose based on the same coin toss code from last time. We then have to make sure to display all the players with their current status.
Enhancements
Next, I want to give a better illustration of how much each player has at any point in time by maybe representing them as bigger or smaller circles on the screen so that as they win or lose, the display shows a bit more dynamic animation than currently with just colours.