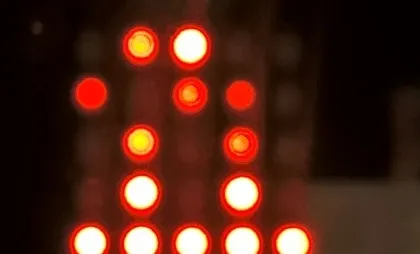
Microbit Ghost Detector
In time for Halloween, here's a ghost detector that uses the microbit temperature sensor to sense changes in room temperature - which we all know from watching supernatural tv shows - is a sure sign that there's a ghost somewhere nearby :)
The code is split into three parts.
Scanner
All good supernatural detector tools need a fancy display so our detector sweeps a line backwards and forwards across the display as if looking for something.
Detector
Our detector is checking for changes in temperature from a reference value and will report when the room temperature drops by one degree or more from the reference.
Program
The main body of the program puts those two elements together and reacts with a ghost image every time it senses a temperature drop.
# ghost-detector.py
from microbit import *
sleep_time = 150
warning_time = 5000
minimum_temperature_difference = 1 # degrees
class SweepScanner:
def __init__(self):
self.direction = 1
self.x = 2
def draw_line(self, brightness):
for y in range(5):
display.set_pixel(self.x, y, brightness)
def scan(self):
self.draw_line(0)
lhs = 0
rhs = 4
if self.x >= rhs or self.x <= lhs:
self.direction = -self.direction
self.x = max(lhs, min(rhs, self.x + self.direction))
self.draw_line(9)
class Detector:
def __init__(self):
self.reset()
def room_is_colder(self):
now_temperature = temperature()
temperature_difference = self.starting_temperature - now_temperature
return temperature_difference >= minimum_temperature_difference
def reset(self):
self.starting_temperature = temperature()
scanner = SweepScanner()
detector = Detector()
while True:
scanner.scan()
sleep(sleep_time)
if detector.room_is_colder():
display.show(Image.GHOST)
sleep(warning_time)
detector.reset()
display.clear()
if button_a.was_pressed():
detector.reset()
Trick
You can use this code to play a trick on someone while telling them a creepy ghost story.
The microbit starts off by reading the current temperature and then monitors the sensor for a colder temperature. It uses this to signal that it thinks a ghost is nearby.
If you start off by holding the microbit in your hand while you tell the beginning of the ghost story, your hand can get it nice and warm. Then, when you put it down somewhere, it will cool down to normal room temperature in a few seconds. That drop in temperature should be enough to trigger the "ghost detector" as you finish your story.
To help with getting the starting temperature just right, you can press the "a" button once you think you have the microbit warm enough to make a difference.