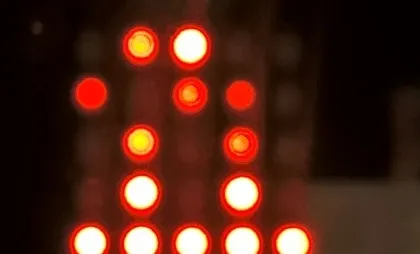
Pythonic Idioms
I always seem to find myself having to look up the "right" way to structure a piece of logic in Python so I thought I would create a little cheatsheet for myself (and anyone else for that matter).
Assignment
We can assign a single value to more than one variable.
a = b = c = d = 0
first = second = 'unknown'
Comparison
I have always done explicit, separate comparisons in an if, probably because I came from a C background. Chaining comparisons together is much more natural for a single value.
if 100 >= value >= 0:
print("value is in the right range!")
Ternary Operators
Slightly more arcane to my mind but reminiscent of ruby syntax, ternary operators work a little different from C-like languages
value = 100 if set_to_maximum else 0
In With the In Crowd
Testing if a value exists in a set of options, or for iterating through a set of values.
exists = value in {'first', 'second', 'third'}
pet_names = ['rover', 'spot', 'fluffy']
for name in pet_names:
print(name)
String Placeholders
print('Happy {celebrant.age} Birthday, {celebrant.name} !'.format(celebrant=person)